Run
Cmd “%windir%\Microsoft.NET\Framework\v4.0.30319\aspnet_regiis.exe
-i”
Reference site for Sitecore and Dot NET. Call at +91-9910045174 for any Sitecore corporate trainings and workshops.
Surendra Sharma
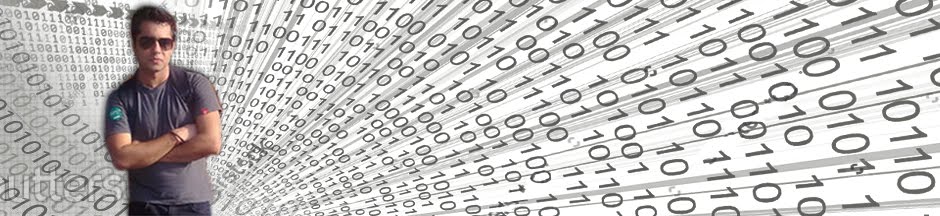
Search This Blog
Wednesday, June 5, 2013
Bind Client Script function
public static void BindClientScript(string
script, Control control) {
ScriptManager.RegisterStartupScript(control, typeof(Page), Guid.NewGuid().ToString(), script, true);
}
How to Get Geocoding Search Results
public static XElement GetGeocodingSearchResults(string address) {
// Use the Google Geocoding service to get information
about the user-entered address
// See
http://code.google.com/apis/maps/documentation/geocoding/index.html for more
info...
var url = String.Format("http://maps.google.com/maps/api/geocode/xml?address={0}&sensor=false",
HttpContext.Current.Server.UrlEncode(address));
// Load the XML into an XElement object (whee, LINQ to
XML!)
var results = XElement.Load(url);
// Check the status
var status = results.Element("status").Value;
if (status != "OK"
&& status != "ZERO_RESULTS")
// Whoops, something else was wrong with the
request...
throw new ApplicationException("There was an error with Google's Geocoding Service:
" + status);
return results;
}
Get Latitude Longitude From Location
public static void GetLatitudeLongitudeFromLocation(string address, out double SelectedLatitude, out
double SelectedLongitude) {
SelectedLatitude = 0;
SelectedLongitude = 0;
string url = "http://maps.googleapis.com/maps/api/geocode/"
+ "xml?keyword=Hotels, +
Australia&address=" + address + "&sensor=false®ion=au";
WebResponse response = null;
try {
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
request.Method = "GET";
response = request.GetResponse();
if (response != null)
{
XPathDocument document = new XPathDocument(response.GetResponseStream());
XPathNavigator navigator =
document.CreateNavigator();
var resultXML = XDocument.Parse(navigator.InnerXml);
if (resultXML != null) {
var geocodeStatus = (from
geocodeResponse in resultXML.Descendants("GeocodeResponse")
select new {
Status = geocodeResponse.Element("status").Value,
}).First();
if (geocodeStatus.Status.Equals("OK"))
{
var geoLocation = (from
Location in resultXML.Descendants("GeocodeResponse").Descendants("geometry").Descendants("location")
select new {
Latitude = Location.Element("lat").Value,
Longitude =
Location.Element("lng").Value,
}).First();
if (geoLocation != null)
{
SelectedLatitude = Convert.ToDouble(geoLocation.Latitude);
SelectedLongitude = Convert.ToDouble(geoLocation.Longitude);
}
}
}
}
}
catch (Exception
ex) {
string
error = ex.Message;
}
finally {
if (response != null)
{
response.Close();
response = null;
}
}
Console.ReadLine();
}
Calculate distance between two points on Earth
public static Double CalculateDistance(Double
latitude1, Double longitude1, Double latitude2, Double
longitude2) {
double theta = longitude1 - longitude2;
double dist = Math.Sin(deg2rad(latitude1))
* Math.Sin(deg2rad(latitude2)) + Math.Cos(deg2rad(latitude1)) * Math.Cos(deg2rad(latitude2)) * Math.Cos(deg2rad(theta));
dist = Math.Acos(dist);
dist
= rad2deg(dist);
dist = dist * 60 * 1.1515;
dist = dist * 1.609344;
return (dist);
}
private static Double deg2rad(Double
deg) {
return (deg * Math.PI
/ 180.0);
}
private static Double rad2deg(Double
rad) {
return (rad / Math.PI
* 180.0);
}
Serialize object to XML
/// <summary>
/// Serialize object to
XML
/// </summary>
/// <param name="instance">Object Instance to be serialized</param>
/// <returns>Respective
XML for specific instace</returns>
public static string SerializeToXml(object
instance) {
string serializedXml = string.Empty;
if (instance != null)
{
StringBuilder sb = new StringBuilder();
StringWriter sw = new StringWriter();
XmlSerializer serializer = new XmlSerializer(instance.GetType(),
"");
serializer.Serialize(sw, instance);
serializedXml = sw.ToString();
}
return serializedXml;
}
Generates Json for specific object instance
Generates
Json for specific object instance
/// <summary>
/// Generates Json for
specific object instance
/// </summary>
/// <param name="instance">Instance to be converted to Json </param>
/// <param name="recursionDepth">Recursion depth optional paramter</param>
/// <returns>Json for
specific object instance</returns>
public static string ToJson(this object instance, int
recursionDepth = 100) {
JavaScriptSerializer serializer = new JavaScriptSerializer();
serializer.RecursionLimit = recursionDepth;
return serializer.Serialize(instance);
}
Subscribe to:
Posts (Atom)